Introduction to ADB Shell Commands
Introduction
A useful feature of Android Debug Bridge (ADB) is the ability to remotely control a device connected either via USB or WiFi using Shell commands e.g.
- adb shell input text "your_text_goes_here“ (sends text as if typed at the keyboard)
- adb shell input swipe 200 900 200 300 (generates a swipe gesture from co-ordinates 200,900 to 200, 300 ( swipe up)
- adb shell input tap 200 300 (generates a screen tap at co-ordinates 200,300
- adb shell input keyevent 4 (generates a keypress event using the table in Appendix A)
The values to use for the co-ordinates in the above commands can be seen easily on the device by enabling the Developer Option ‘Pointer Location’ which will then show a pointer trace and the current X,Y screen co-ordinates in the top line of the display:
Use Case
Combining the adb shell commands together will allow a device to be remotely controlled /configured via a PC and a command script. This allows automatic configuration of multiple devices connected to one PC via ADB which can significantly reduce the staging time required for either:
a) devices such as the ET1 which are not supporting the full MX functionality
b) configuring features which are not covered by the existing MX functionality e.g. Wireless hotspot
Note a couple of disadvantages of using this method for staging devices:
a) the settings are not persistent i.e. if the device is Enterprise Reset in the field, it will require restaging using the same method (or manually)
b) the UI of the device can change between OS versions e.g. some of the Settings screens for JellyBean devices are different to KitKat and it may be necessary to have two script files , one for each OS version.
A simple way to sequence ADB shell commands is to put them into a batch file e.g.
a) Configure French language settings on a TC55 JellyBean OS device:
adb shell am start -a android.intent.action.MAIN -n com.android.settings/.LanguageSettings
rem select language option
adb shell input tap 200 160
rem select UK - swipe up
adb shell input swipe 100 700 100 200
rem select France
adb shell input tap 200 780
rem return to Home Screen
adb shell input keyevent 3
b) Configure WiFi HotSpot on a TC 55 JB OS
adb shell am start -a android.intent.action.MAIN -n com.android.settings/.wifi.WifiSettings
adb shell input tap 40 80
rem select More
adb shell input tap 240 400
rem Enable Hotspot
adb shell input tap 240 320
adb shell input tap 240 320
adb shell input tap 240 700
adb shell input tap 240 600
rem delete existing passkey using backspace
adb shell input keyevent 67
adb shell input keyevent 67
adb shell input keyevent 67
adb shell input keyevent 67
adb shell input keyevent 67
adb shell input keyevent 67
adb shell input keyevent 67
adb shell input keyevent 67
adb shell input keyevent 67
adb shell input keyevent 67
adb shell input keyevent 67
adb shell input keyevent 67
adb shell input keyevent 67
rem set new passkey 'zebra'
adb shell input text zebra
adb shell input keyevent 4
adb shell input tap 240 770
ADB Over WiFi
ADB commands will work over USB or WiFi connections . To configure a WiFi connection, the device must first be connected via USB and configured for wireless via the following commands:
adb tcpip 5555
adb connect 192.168.0.101:5555
(Replace 192.168.0.101 with the IP address that is actually assigned to your device)
At this point the USB cable can be removed and commands will be sent wirelessly. Note that you can find the IP address of a device by either:
- a) Go into Settings/About phone/Status and check the IP address field
- b) Execute the following command via adb:
adb shell ip -f inet addr show wlan0
To reset the ADB connection to USB , type :
adb usb
Reconnect the USB cable and the device should be recognized again .
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
Appendix A – Keycode table
adb shell input keyevent
0 --> "KEYCODE_UNKNOWN"
1 --> "KEYCODE_MENU"
2 --> "KEYCODE_SOFT_RIGHT"
3 --> "KEYCODE_HOME"
4 --> "KEYCODE_BACK"
5 --> "KEYCODE_CALL"
6 --> "KEYCODE_ENDCALL"
7 --> "KEYCODE_0"
8 --> "KEYCODE_1"
9 --> "KEYCODE_2"
10 --> "KEYCODE_3"
11 --> "KEYCODE_4"
12 --> "KEYCODE_5"
13 --> "KEYCODE_6"
14 --> "KEYCODE_7"
15 --> "KEYCODE_8"
16 --> "KEYCODE_9"
17 --> "KEYCODE_STAR"
18 --> "KEYCODE_POUND"
19 --> "KEYCODE_DPAD_UP"
20 --> "KEYCODE_DPAD_DOWN"
21 --> "KEYCODE_DPAD_LEFT"
22 --> "KEYCODE_DPAD_RIGHT"
23 --> "KEYCODE_DPAD_CENTER"
24 --> "KEYCODE_VOLUME_UP"
25 --> "KEYCODE_VOLUME_DOWN"
26 --> "KEYCODE_POWER"
27 --> "KEYCODE_CAMERA"
28 --> "KEYCODE_CLEAR"
29 --> "KEYCODE_A"
30 --> "KEYCODE_B"
31 --> "KEYCODE_C"
32 --> "KEYCODE_D"
33 --> "KEYCODE_E"
34 --> "KEYCODE_F"
35 --> "KEYCODE_G"
36 --> "KEYCODE_H"
37 --> "KEYCODE_I"
38 --> "KEYCODE_J"
39 --> "KEYCODE_K"
40 --> "KEYCODE_L"
41 --> "KEYCODE_M"
42 --> "KEYCODE_N"
43 --> "KEYCODE_O"
44 --> "KEYCODE_P"
45 --> "KEYCODE_Q"
46 --> "KEYCODE_R"
47 --> "KEYCODE_S"
48 --> "KEYCODE_T"
49 --> "KEYCODE_U"
50 --> "KEYCODE_V"
51 --> "KEYCODE_W"
52 --> "KEYCODE_X"
53 --> "KEYCODE_Y"
54 --> "KEYCODE_Z"
55 --> "KEYCODE_COMMA"
56 --> "KEYCODE_PERIOD"
57 --> "KEYCODE_ALT_LEFT"
58 --> "KEYCODE_ALT_RIGHT"
59 --> "KEYCODE_SHIFT_LEFT"
60 --> "KEYCODE_SHIFT_RIGHT"
61 --> "KEYCODE_TAB"
62 --> "KEYCODE_SPACE"
63 --> "KEYCODE_SYM"
64 --> "KEYCODE_EXPLORER"
65 --> "KEYCODE_ENVELOPE"
66 --> "KEYCODE_ENTER"
67 --> "KEYCODE_DEL"
68 --> "KEYCODE_GRAVE"
69 --> "KEYCODE_MINUS"
70 --> "KEYCODE_EQUALS"
71 --> "KEYCODE_LEFT_BRACKET"
72 --> "KEYCODE_RIGHT_BRACKET"
73 --> "KEYCODE_BACKSLASH"
74 --> "KEYCODE_SEMICOLON"
75 --> "KEYCODE_APOSTROPHE"
76 --> "KEYCODE_SLASH"
77 --> "KEYCODE_AT"
78 --> "KEYCODE_NUM"
79 --> "KEYCODE_HEADSETHOOK"
80 --> "KEYCODE_FOCUS"
81 --> "KEYCODE_PLUS"
82 --> "KEYCODE_MENU"
83 --> "KEYCODE_NOTIFICATION"
84 --> "KEYCODE_SEARCH"
85 --> "TAG_LAST_KEYCODE"
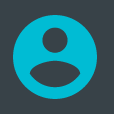
Anonymous (not verified)
1 Replies
Is it possible to use ADB to emulate barcode scanning intents? I've been following the guide <a href="/community/android/android-forums/android-blogs/blog/2014/11/06/scanning-barcodes-in-your-xamarin-android-app">here</a> for developing an app that uses my TC70's barcode reader but want a way of emulating the scan for debugging on a different device that doesn't have the TC70's scanner.